Simple example for VueJS Search Filter
in VueJS
Search is an extremely important component in every website that contains many categories and components. Especially the site about e-commerce, blog, news, etc. All need the search function to select information.
This article applies to the quick search of data in the array that is returned as soon as you enter your search keywords, below I have written how to call the API that returns the datas array and assuming an array datas is available.
Simple example for VueJS Search Filter
First, we assume that there is an API that returns data, including post title and article category name:
datas: [
{ title:”Add weather and time zone information to your website from free API”, category:”VueJS” },
{ title:”How to import data from excel file, csv into the database and export back”, category:”PHP-MySQL” },
{ title:”PHP – MVC CRUD and Connect to MySQL using PDO”, category:”PHP-MySQL” },
{ title:”Automatically backup MySQL database with Batch file on Windows”, category:”PHP-MySQL” },
{ title:”Add and Remove input textbox with Jquery, Javascript”, category:”Jquery-Javascript” },
{ title:”Customizable multi-type, multi-y axis code ChartJS – Part 2″, category:”Jquery-Javascript” },
{ title:”Customizable multi-type, multi-y axis code ChartJS – Part 1″, category:”Jquery-Javascript” },
];
If you have VueJS installed, that’s great, but just want to test quickly without installation, add the VueJS library as below, and add Bootstrap to use it.
<script src=”https://cdn.jsdelivr.net/npm/vue@2.6.12″></script>
<link rel=”stylesheet” href=”https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css”>
About template.vue
– You need an input box to enter the keyword search, a loading icon shows the time it takes to show dataư
– resultQuery: If there is datas, the data will be retrieved in the for loop
<template>
<div class=”col-md-12 text-center”>
<div class=”col-md-4 p-0″>
<input type=”text” v-model=”quickSearch” placeholder=”Quick search…” class=”form-control”>
</div>
<p v-if=”loading” class=”text-center”><img :src=”‘https://softdownloadfree.com/uploads/images/loading-icon.gif'” alt=”loading” width=”35px”/></p><div class=”text-left” v-if=”resultQuery”>
<div class=”col-md-12 mb-1″ style=”display:inline-block” v-for=”item in resultQuery”>
<h3 v-if=”item.title”>{{ item.title }}</h3>
</div>
</div>
</div>
</template>
About resultQuery data processing:
– If you have not entered the search keyword, the data will display all the values in the datas array which were retrieved (return this.datas).
– When entering search keywords, you can search only by title or multiple arguments (VueJS search filter multiple arguments). Below is the search by title and category, and is not case sensitive.
<script type=”text/javascript”>
export default {
data () {
return {
error: null,
loading: true,
datas: [
{ title:”Add weather and time zone information to your website from free API”, category:”VueJS” },
{ title:”How to import data from excel file, csv into the database and export back”, category:”PHP-MySQL” },
{ title:”PHP – MVC CRUD and Connect to MySQL using PDO”, category:”PHP-MySQL” },
{ title:”Automatically backup MySQL database with Batch file on Windows”, category:”PHP-MySQL” },
{ title:”Add and Remove input textbox with Jquery, Javascript”, category:”Jquery-Javascript” },
{ title:”Customizable multi-type, multi-y axis code ChartJS – Part 2″, category:”Jquery-Javascript” },
{ title:”Customizable multi-type, multi-y axis code ChartJS – Part 1″, category:”Jquery-Javascript” },
];
quickSearch: null,
}
},
computed: {
resultQuery() {
if (this.quickSearch) {
return this.datas.filter((item)=>{
return this.quickSearch.toLowerCase().split(‘ ‘).every(v => item.title.toLowerCase().includes(v) || item.category.toLowerCase().includes(v))
})
} else {
return this.datas;
}
},
}
}
</script>
At this point, we have completed the simple vuejs search filter with the given data.
If you get the data returned by the API, you should use axios to do this.
The beforeRouteEnter and beforeRouteUpdate functions are used to load the priority data as soon as the page is loaded.
<script type=”text/javascript”>
import axios from ‘axios’;
const getData = (page, callback) => {
const params = { page };
axios
.get(‘/api/post’, { params })
.then(response => {
callback(null, response.data);
})
.catch(error => {
callback(error, response);
});
};export default {
data () {
return {
error: null,
loading: true,
datas: null,
quickSearch: null,
}
},
computed: {
resultQuery() {
if (this.quickSearch) {
return this.datas.filter((item)=>{
return this.quickSearch.toLowerCase().split(‘ ‘).every(v => item.title.toLowerCase().includes(v) || item.category.toLowerCase().includes(v))
})
} else {
return this.datas;
}
},
beforeRouteEnter(to, from, next) {
getData(to.query.page, (err, results) => {
next(vm => vm.setData(err, results));
})
},
beforeRouteUpdate(to, from, next) {
this.loading = true;
this.results = null;
getData(to.query.page, (err, results) => {
this.setData(err, results);
next();
})
},
methods: {
setData(err, {results}) {
this.loading = true;
if (err) {
this.err = err.toString();
} else {
this.loading = false;
this.datas = results;
}
},
}
}
}
</script>
So we have completed the quick search function with multiple parameters using VueJS
You can refer to the results at the home page: https://softdownloadfree.com or site category page https://softdownloadfree.com/category/vuejs/
Thank you for reading the article
Related posts
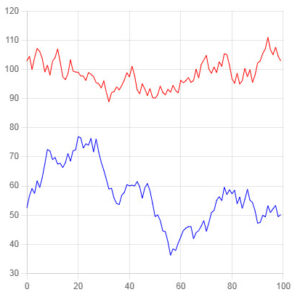
Chart js animation example
Jquery Javascript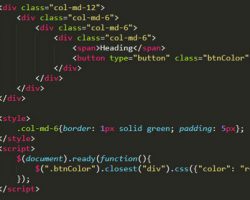
Jquery & Javascript Code snippet might be helpful for you
Jquery Javascript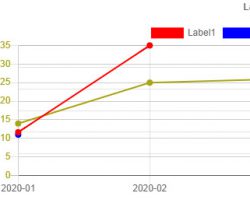
Your comment